1、添加依赖
1 2 3 4 5 6 7 8 9 10
| <dependency> <groupId>ch.ethz.ganymed</groupId> <artifactId>ganymed-ssh2</artifactId> <version>262</version> </dependency> <dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.6</version> </dependency>
|
2、Api说明
- 首先构造一个连接器,传入一个需要登陆的ip地址;
1
| Connection conn = new Connection(ipAddr);
|
- 模拟登陆目的服务器,传入用户名和密码;
1
| boolean isAuthenticated = conn.authenticateWithPassword(userName, passWord);
|
它会返回一个布尔值,true 代表成功登陆目的服务器,否则登陆失败。
- 打开一个session,执行你需要的linux 脚本命令;
1 2
| Session session = conn.openSession(); session.execCommand(“ifconfig”);
|
- 接收目标服务器上的控制台返回结果,读取br中的内容;
1 2
| InputStream stdout = new StreamGobbler(session.getStdout()); BufferedReader br = new BufferedReader(new InputStreamReader(stdout));
|
- 得到脚本运行成功与否的标志 :0-成功 非0-失败
1
| System.out.println(“ExitCode: ” + session.getExitStatus());
|
- 关闭session和connection
1 2
| session.close(); conn.close();
|
Tips:
- 通过第二部认证成功后当前目录就位于/home/username/目录之下,你可以指定脚本文件所在的绝对路径,或者通过cd导航到脚本文件所在的目录,然后传递执行脚本所需要的参数,完成脚本调用执行。
- 执行脚本以后,可以获取脚本执行的结果文本,需要对这些文本进行正确编码后返回给客户端,避免乱码产生。
- 如果你需要执行多个linux控制台脚本,比如第一个脚本的返回结果是第二个脚本的入参,你必须打开多个Session,也就是多次调用
Session sess = conn.openSession();,使用完毕记得关闭就可以了。
3. 实例:工具类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93
| public class SSHTool {
private Connection conn; private String ipAddr; private Charset charset = StandardCharsets.UTF_8; private String userName; private String password;
public SSHTool(String ipAddr, String userName, String password, Charset charset) { this.ipAddr = ipAddr; this.userName = userName; this.password = password; if (charset != null) { this.charset = charset; } }
private boolean login() { conn = new Connection(ipAddr);
try { conn.connect(); return conn.authenticateWithPassword(userName, password); } catch (IOException e) { e.printStackTrace(); return false; } }
public StringBuilder exec(String cmds) throws IOException { InputStream in = null; StringBuilder result = new StringBuilder(); try { if (this.login()) { Session session = conn.openSession(); session.execCommand(cmds); in = session.getStdout(); result = this.processStdout(in, this.charset); conn.close(); } } finally { if (null != in) { in.close(); } } return result; }
public StringBuilder processStdout(InputStream in, Charset charset) throws FileNotFoundException { byte[] buf = new byte[1024]; StringBuilder sb = new StringBuilder();
try { int length; while ((length = in.read(buf)) != -1) {
sb.append(new String(buf, 0, length)); } } catch (IOException e) { e.printStackTrace(); } return sb; }
public static void main(String[] args) throws IOException { SSHTool tool = new SSHTool("192.168.100.40", "root", "123456", StandardCharsets.UTF_8); StringBuilder exec = tool.exec("bash /root/test12345.sh"); System.out.println(exec); } }
|
4、测试脚本
输出结果
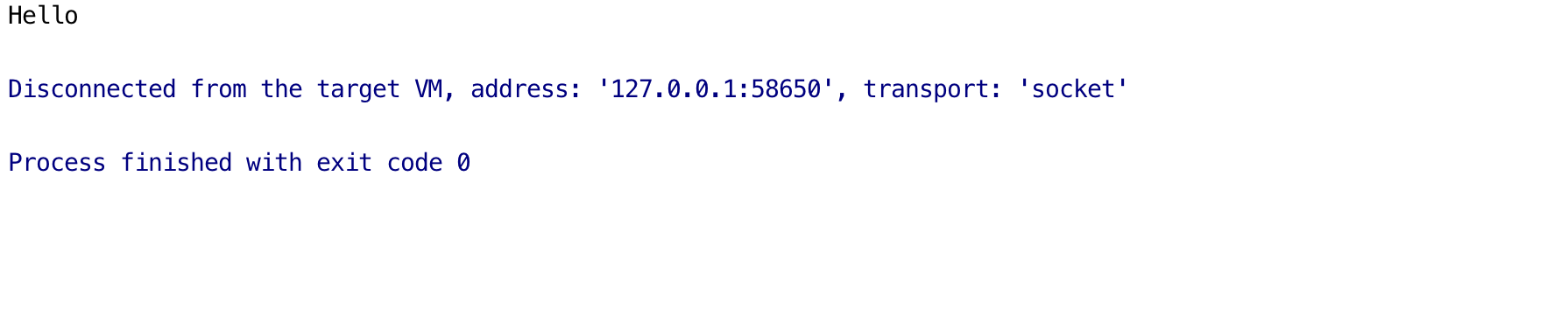